GitHub Copilot Labsを使ってコードをいい感じに直す
はじめに
先日社内でGitHub Copilot for Businessが正式導入され、開発現場でも活用が進んできています。
(GitHub Copilot for Businessに関する記事はこちら↓)
その過程でCopilotの支援機能としてGitHub Copilot LabsというVSCode拡張を見つけたので、早速触ってみることにしました。
公式サイトはこちら。
(この拡張は2022/3/29に公開されたようなので、執筆時点でちょうど1年ですね。)
インストール
VSCodeの拡張からGitHub Copilot Labsを選択してインストールしましょう。
この拡張はGithub Copilotの使用を前提としているので、事前にGithubアカウントでCopilotが使える状態にしておく必要があります。
機能一覧
GitHub Copilot Labsには以下の機能があります。
- EXPLAIN
- LANGUAGE TRANSLATION
- BRUSHES
- READABLE
- ADD TYPES
- FIX BUG
- DEBUG
- CLEAN
- LIST STEPS
- MAKE ROBUST
- CHUNK
- DOCUMENT
- CUSTOM
- TEST GENERATION
基本的な使い方
エディタ上でコードを選択し、GitHub Copilot Labs上で Ask Copilot
や特定の機能のボタンをクリックするだけです。
下の画像ではEXPLAIN
を実行して、この関数の説明を作成しています。
サンプルコードで色々やってみる
今回はChatGPT君に作成してもらったコードを例にGitHub Copilot Labsの機能をいくつか使ってみましょう。
function calculateTotalPrice(price, quantity, taxRate): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount;
return totalPrice;
}
EXPLAIN (コードの説明や使用例などの作成)
選択した機能に応じてドキュメントを作成してくれます。
/* Here is the explanation for the code above:
1. The function calculateTotalPrice has three parameters:
- price which is a number
- quantity which is a number
- taxRate which is a number
2. The function returns a number.
3. The function calculates the total price by multiplying the price and quantity.
4. It then calculates the tax amount by multiplying the total with the tax rate.
5. It then calculates the total price by subtracting the tax amount from the total.
6. It returns the total price. */
/* This is an example for calling this function:
* let price = 5;
* let quantity = 10;
* let taxRate = 0.25;
* let totalPrice = calculateTotalPrice(price, quantity, taxRate); */
LANGUAGE TRANSLATION (他言語への変換)
コードを指定した言語に変換してくれます。
def calculate_total_price(price, quantity, tax_rate):
total = price * quantity
tax_amount = total * tax_rate
total_price = total - tax_amount
return total_price
BRUSHES (リファクタリング)
READABLE (コメント追加)
コードの可読性を上げるためのコメントを追加してくれます。
function calculateTotalPrice(price, quantity, taxRate): number {
let total = price * quantity; // total price of the item without tax
let taxAmount = total * taxRate; // tax amount
let totalPrice = total + taxAmount; // total price of the item with tax
return totalPrice;
}
ADD TYPES (型追加)
型指定を追加してくれます。
function calculateTotalPrice(price: number, quantity: number, taxRate: number): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount;
return totalPrice;
}
FIX BUG (バグ修正)
いい感じにバグ修正してくれます。
function calculateTotalPrice(price, quantity, taxRate): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total - taxAmount; // + を - にしてバグを挿入
return totalPrice;
}
function calculateTotalPrice(price, quantity, taxRate): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount; // +に正しく修正
return totalPrice;
}
DEBUG (デバッグコードの追加)
開発時に有用なデバッグコードを追加してくれます。
function calculateTotalPrice(price, quantity, taxRate): number {
console.log('price:', price);
console.log('quantity:', quantity);
console.log('taxRate:', taxRate);
let total = price * quantity;
console.log('total:', total);
let taxAmount = total * taxRate;
console.log('taxAmount:', taxAmount);
let totalPrice = total + taxAmount;
console.log('totalPrice:', totalPrice);
return totalPrice;
}
CLEAN (デバッグコードの削除)
DEBUGで追加したコードを削除してくれます。
LIST STEPS : コメント追加
処理ステップ毎の詳細なコメントを追加してくれます。
function calculateTotalPrice(price, quantity, taxRate): number {
// Multiply the price by the quantity to get the subtotal
let total = price * quantity;
// Multiply the subtotal by the tax rate to get the tax amount
let taxAmount = total * taxRate;
// Add the tax amount to the subtotal to get the total price
let totalPrice = total + taxAmount;
return totalPrice;
}
MAKE ROBUST (堅牢化)
引数のチェックやエラーハンドリングを追加してくれます。
function calculateTotalPrice(price, quantity, taxRate): number {
// handle price
if (typeof price !== 'number') {
throw new Error('Price must be a number');
}
if (price < 0) {
throw new Error('Price must be positive');
}
// handle quantity
if (typeof quantity !== 'number') {
throw new Error('Quantity must be a number');
}
if (quantity < 0) {
throw new Error('Quantity must be positive');
}
// handle taxRate
if (typeof taxRate !== 'number') {
throw new Error('Tax rate must be a number');
}
if (taxRate < 0) {
throw new Error('Tax rate must be positive');
}
// calculate total
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount;
return totalPrice;
}
CHUNK (細分化)
関数の責務を細分化してくれます。
function calculateTotalPrice(price: number, quantity: number, taxRate: number): number {
let total = calculateTotal(price, quantity);
let taxAmount = calculateTaxAmount(total, taxRate);
let totalPrice = calculateTotalPrice(total, taxAmount);
return totalPrice;
}
function calculateTotal(price: number, quantity: number): number {
return price * quantity;
}
function calculateTaxAmount(total: number, taxRate: number): number {
return total * taxRate;
}
function calculateTotalPrice(total: number, taxAmount: number): number {
return total + taxAmount;
}
DOCUMENT (ドキュメント追加)
コードの詳細な説明文を追加してくれます。
// This code calculates the total price of a product. It takes in the price, quantity, and tax rate of the product.
// The function returns the total price of the product.
// The total price is calculated by multiplying the price by the quantity, then adding the tax amount to the total.
// The tax amount is calculated by multiplying the total by the tax rate.
function calculateTotalPrice(price: number, quantity: number, taxRate: number): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount;
return totalPrice;
}
CUSTOM (独自のコード修正)
Copilotに指示を出すのと同じように、独自の修正指示が出せます。
change function name to more suitable name
と入力したところ、関数名をcalculateTotalPrice
-> calculateTotalPriceWithTax
に変更してくれました。
function calculateTotalPriceWithTax(price, quantity, taxRate): number {
let total = price * quantity;
let taxAmount = total * taxRate;
let totalPrice = total + taxAmount;
return totalPrice;
}
TEST GENERATION (テストコード作成)
テストコードを作成してくれます。
describe('test foo', function() {
it('test foo.calculateTotalPriceWithTax', function(done) {
let price = 100;
let quantity = 2;
let taxRate = 0.1;
let expected = 220;
let actual = foo.calculateTotalPriceWithTax(price, quantity, taxRate);
assert.equal(actual, expected);
done();
})
})
まとめ
一部の機能はCopilot本体でも実現できるかもしれませんが、機能がシンプルにまとまっているので併用してみても良さそうです。
引き続き開発体験の向上を目指して便利ツールを探していきたいと思います。
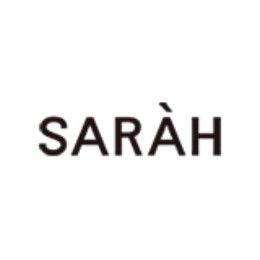
SARAHでは一皿に特化したごはん情報の投稿・配信・収集・解析するサービスをtoC,toBと多角的に展開しています。アプリ / Web / SaaS / データサイエンス を最新の環境と技術で広く運用します。 技術スタック詳細はこちら→ stackshare.io/companies/sarah-inc
Discussion