Open5
Deno で Lambda 関数をローカル開発する方法について
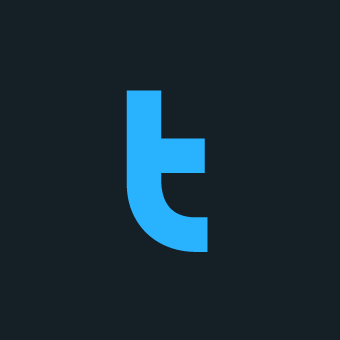
最初にやるコト
Deno の開発環境を準備する。
$ deno init --unstable deno-with-lambda
✅ Project initialized
Run these commands to get started
cd deno-with-lambda
# Run the program
deno run main.ts
# Run the program and watch for file changes
deno task dev
# Run the tests
deno test
とりあえず動くかチェックする
$ cd deno-with-lambda
$ deno run main.ts
Add 2 + 3 = 5
$ deno task dev
Task dev deno run --watch main.ts
Watcher Process started.
Add 2 + 3 = 5
Watcher Process finished. Restarting on file change...
良さそう。
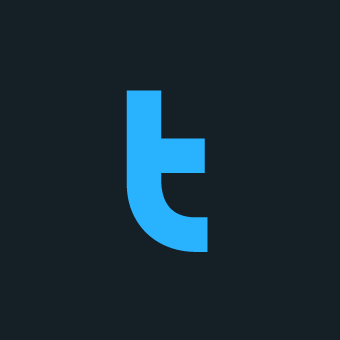
Hello World
するために直していく
Lambda で 先ほど作った main.ts
を修正していく。
import {
Context,
APIGatewayProxyEventV2,
APIGatewayProxyResultV2,
} from "https://deno.land/x/lambda@1.45.3/mod.ts";
export async function handler(
event: APIGatewayProxyEventV2,
context: Context
): Promise<APIGatewayProxyResultV2> {
const result = await fetch("https://api.github.com/users/tbaba");
const json = await result.json();
return {
statusCode: 200,
body: JSON.stringify({
message: JSON.stringify(json),
event,
context,
}),
};
}
要するに、「 GitHub からぼくの情報取ってきて返す」というシンプルな API 。
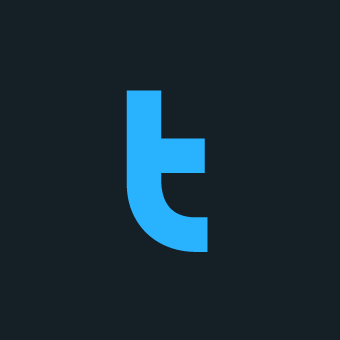
ローカルで動かしてみるための Docker イメージを作る
公式が、Deno を Lambda で動かすためのベースを作ってくれているので、これを参考にしながら作っていく。
$ touch Dockerfile
$ ls
Dockerfile deno.json deno.lock main.ts
FROM denoland/deno-lambda:1.45.3
COPY main.ts .
RUN deno cache main.ts
CMD ["main.handler"]
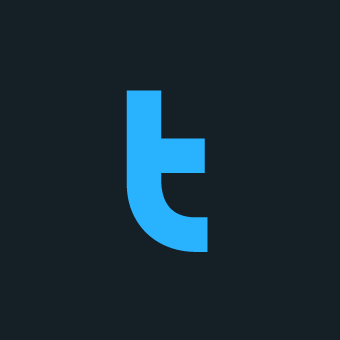
試す
実際に動かしてみる。
$ docker build -t deno-with-lambda:test .
[+] Building 2.9s (9/9) FINISHED docker:desktop-linux
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 131B 0.0s
=> [internal] load metadata for docker.io/denoland/deno-lambda:1.45.3 2.2s
=> [auth] denoland/deno-lambda:pull token for registry-1.docker.io 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load build context 0.0s
=> => transferring context: 526B 0.0s
=> CACHED [1/3] FROM docker.io/denoland/deno-lambda:1.45.3@sha256:5d086564eecfef7378d86ce582780634e78b7f4c80d296e1b8e75083f8dd18f8 0.0s
=> [2/3] COPY main.ts . 0.0s
=> [3/3] RUN deno cache main.ts 0.5s
=> exporting to image 0.0s
=> => exporting layers 0.0s
=> => writing image sha256:3edfff3ed3db8df611d7aaa36984f25f1860210c4fa51ba4bb9627bb6b42e2c7 0.0s
=> => naming to docker.io/library/deno-with-lambda:test 0.0s
View build details: docker-desktop://dashboard/build/desktop-linux/desktop-linux/bxf0zzbqjywaogfxyqehjmf6v
What's next:
View a summary of image vulnerabilities and recommendations → docker scout quickview
$ docker run -p 9000:8080 deno-with-lambda:test
WARNING: The requested image's platform (linux/amd64) does not match the detected host platform (linux/arm64/v8) and no specific platform was requested
24 Jul 2024 04:57:15,676 [INFO] (rapid) exec '/var/runtime/bootstrap' (cwd=/var/task, handler=)
この状態になったら、別のコンソールを開くなりして、リクエストを送信してみる。その際に一応リクエストボディを作っておかないとエラーが返ってくるので注意。
$ curl -XPOST "http://localhost:9000/2015-03-31/functions/function/invocations" -d "{}"
{"statusCode":200,"body":"{\"message\":\"{\\\"login\\\":\\\"tbaba\\\",\\\"id\\\":234799,\\\"node_id\\\":\\\"MDQ6VXNlcjIzNDc5OQ==\\\",\\\"avatar_url\\\":\\\"https://avatars.githubusercontent.com/u/234799?v=4\\\",\\\"gravatar_id\\\":\\\"\\\",\\\"url\\\":\\\"https://api.github.com/users/tbaba\\\",\\\"html_url\\\":\\\"https://github.com/tbaba\\\",\\\"followers_url\\\":\\\"https://api.github.com/users/tbaba/followers\\\",\\\"following_url\\\":\\\"https://api.github.com/users/tbaba/following{/other_user}\\\",\\\"gists_url\\\":\\\"https://api.github.com/users/tbaba/gists{/gist_id}\\\",\\\"starred_url\\\":\\\"https://api.github.com/users/tbaba/starred{/owner}{/repo}\\\",\\\"subscriptions_url\\\":\\\"https://api.github.com/users/tbaba/subscriptions\\\",\\\"organizations_url\\\":\\\"https://api.github.com/users/tbaba/orgs\\\",\\\"repos_url\\\":\\\"https://api.github.com/users/tbaba/repos\\\",\\\"events_url\\\":\\\"https://api.github.com/users/tbaba/events{/privacy}\\\",\\\"received_events_url\\\":\\\"https://api.github.com/users/tbaba/received_events\\\",\\\"type\\\":\\\"User\\\",\\\"site_admin\\\":false,\\\"name\\\":\\\"Tatsuro Baba\\\",\\\"company\\\":\\\"@grooves \\\",\\\"blog\\\":\\\"\\\",\\\"location\\\":\\\"Tokyo, Japan\\\",\\\"email\\\":null,\\\"hireable\\\":null,\\\"bio\\\":\\\"Web Application Developer.\\\",\\\"twitter_username\\\":null,\\\"public_repos\\\":91,\\\"public_gists\\\":30,\\\"followers\\\":33,\\\"following\\\":14,\\\"created_at\\\":\\\"2010-04-01T08:42:08Z\\\",\\\"updated_at\\\":\\\"2024-06-22T14:27:08Z\\\"}\",\"event\":{},\"context\":{\"functionName\":\"test_function\",\"functionVersion\":\"$LATEST\",\"invokedFunctionArn\":\"arn:aws:lambda:us-east-1:012345678912:function:test_function\",\"memoryLimitInMB\":\"3008\",\"awsRequestId\":\"515da620-87f6-47ce-991f-fb3f3b08551e\",\"logGroupName\":\"/aws/lambda/Functions\",\"logStreamName\":\"$LATEST\"}}"}%