Flutter | Banner Adと重複しないためのFloating Action Button (FAB)の位置変更メモ
この画像のように、BannerAdを一番下に表示する場合、そのままのFloating Action Button (FAB)ではBannerAdと重なってしまう。そこで、BannerAdに対してFABのマージンを設定したい。
FABの位置変更には2種類ある。
1つは、floatingActionButtonLocationにより、基準位置を変更する方法。
もう1つは、FloatingActionButton WidgetをPaddingで囲う方法。
floatingActionButtonLocationによる位置変更
ScaffoldのfloatingActionButtonLocationオプションに、FloatingActionButtonLocationを指定する。appBarとFABをドッキングさせたり、左下の表示する等、絶対的な基準位置を変更する際に使用する。今回は、デフォルトの位置のまま、BannerAdを避けるよう上にFABを移動したいだけのため使用しない。
class Test extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
body: Column(),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
);
}
}
Flutterの「FloatingActionButtonLocation class」がわかりやすい。
参考までに、FloatingActionButtonLocationのabstract classを載せておく。
abstract class FloatingActionButtonLocation {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const FloatingActionButtonLocation();
/// Start-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartTop] and set [FloatingActionButton.mini] to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 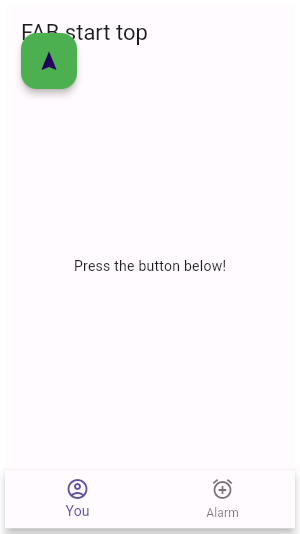
static const FloatingActionButtonLocation startTop = _StartTopFabLocation();
/// Start-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], optimized for mini floating
/// action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 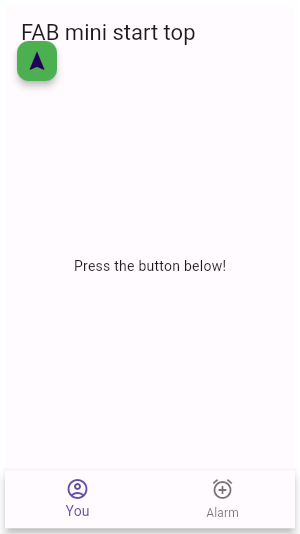
static const FloatingActionButtonLocation miniStartTop = _MiniStartTopFabLocation();
/// Centered [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 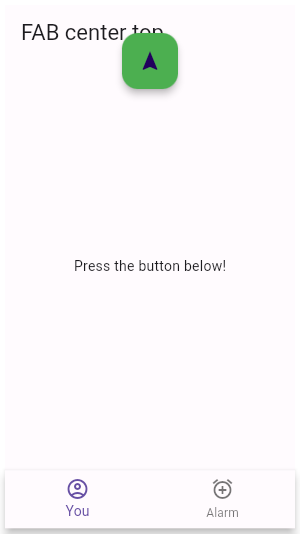
static const FloatingActionButtonLocation centerTop = _CenterTopFabLocation();
/// Centered [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], intended to be used with
/// [FloatingActionButton.mini] set to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 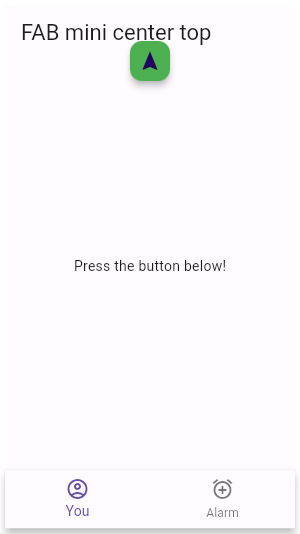
static const FloatingActionButtonLocation miniCenterTop = _MiniCenterTopFabLocation();
/// End-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body].
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndTop] and set [FloatingActionButton.mini] to true.
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 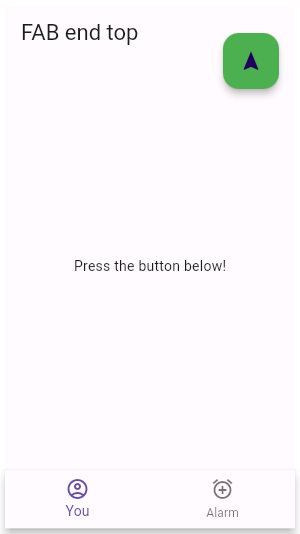
static const FloatingActionButtonLocation endTop = _EndTopFabLocation();
/// End-aligned [FloatingActionButton], floating over the transition between
/// the [Scaffold.appBar] and the [Scaffold.body], optimized for mini floating
/// action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a top [AppBar]
/// or that use a [SliverAppBar] in the scaffold body itself.
///
/// 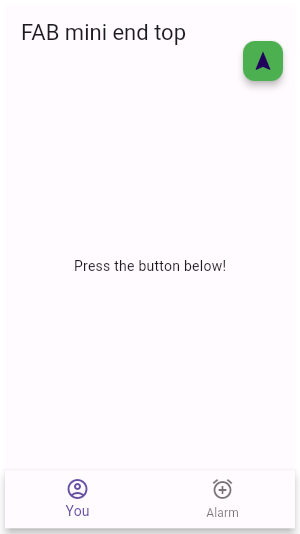
static const FloatingActionButtonLocation miniEndTop = _MiniEndTopFabLocation();
/// Start-aligned [FloatingActionButton], floating at the bottom of the screen.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartFloat] and set [FloatingActionButton.mini] to true.
///
/// 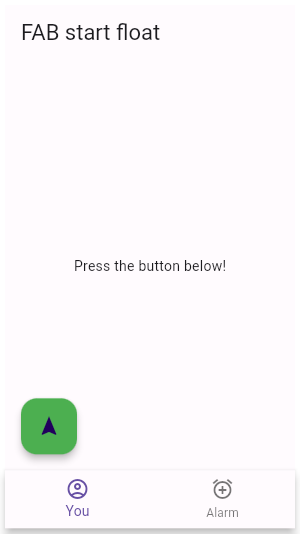
static const FloatingActionButtonLocation startFloat = _StartFloatFabLocation();
/// Start-aligned [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// Compared to [FloatingActionButtonLocation.startFloat], floating action
/// buttons using this location will move horizontally _and_ vertically
/// closer to the edges, by [kMiniButtonOffsetAdjustment] each.
///
/// 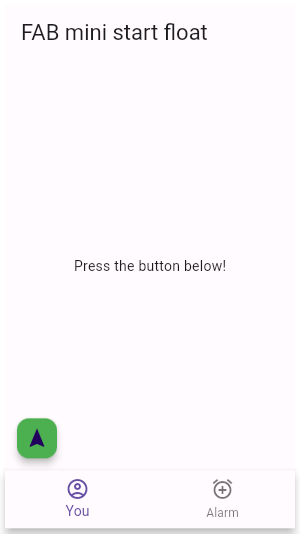
static const FloatingActionButtonLocation miniStartFloat = _MiniStartFloatFabLocation();
/// Centered [FloatingActionButton], floating at the bottom of the screen.
///
/// To position a mini floating action button, use [miniCenterFloat] and
/// set [FloatingActionButton.mini] to true.
///
/// 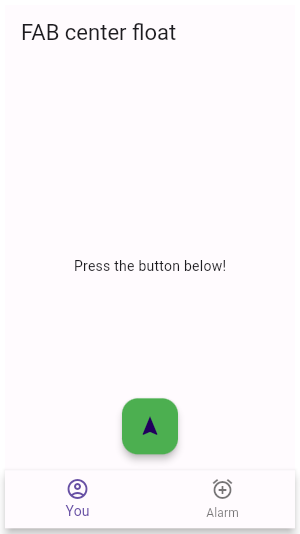
static const FloatingActionButtonLocation centerFloat = _CenterFloatFabLocation();
/// Centered [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align horizontally with
/// the locations [FloatingActionButtonLocation.miniStartFloat]
/// and [FloatingActionButtonLocation.miniEndFloat].
///
/// Compared to [FloatingActionButtonLocation.centerFloat], floating action
/// buttons using this location will move vertically down
/// by [kMiniButtonOffsetAdjustment].
///
/// 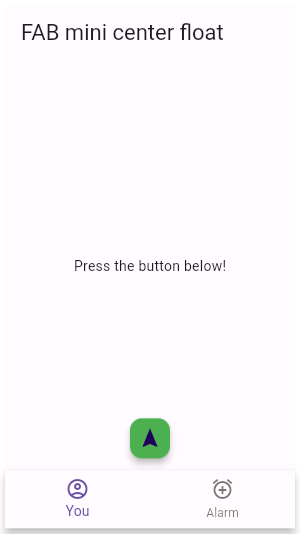
static const FloatingActionButtonLocation miniCenterFloat = _MiniCenterFloatFabLocation();
/// End-aligned [FloatingActionButton], floating at the bottom of the screen.
///
/// This is the default alignment of [FloatingActionButton]s in Material applications.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndFloat] and set [FloatingActionButton.mini] to true.
///
/// 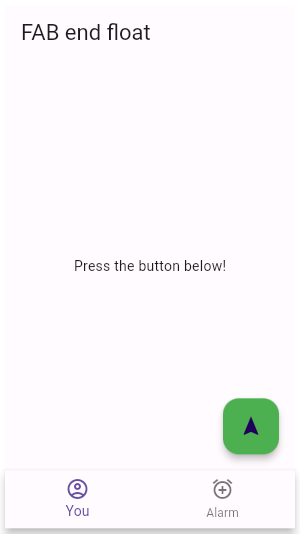
static const FloatingActionButtonLocation endFloat = _EndFloatFabLocation();
/// End-aligned [FloatingActionButton], floating at the bottom of the screen,
/// optimized for mini floating action buttons.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// Compared to [FloatingActionButtonLocation.endFloat], floating action
/// buttons using this location will move horizontally _and_ vertically
/// closer to the edges, by [kMiniButtonOffsetAdjustment] each.
///
/// 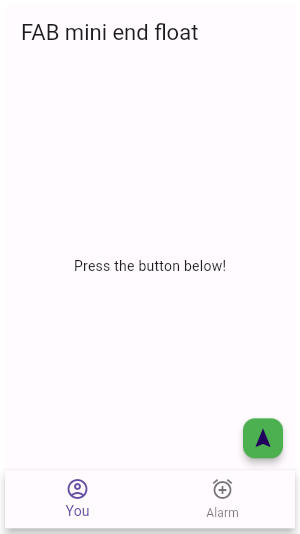
static const FloatingActionButtonLocation miniEndFloat = _MiniEndFloatFabLocation();
/// Start-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.leading] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniStartDocked] and set [FloatingActionButton.mini] to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 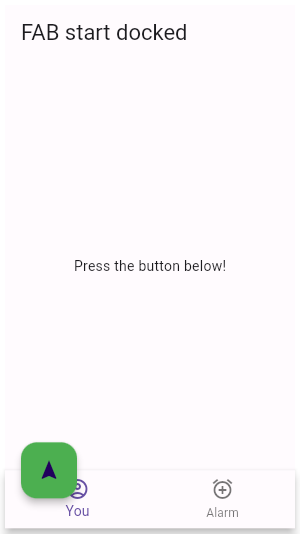
static const FloatingActionButtonLocation startDocked = _StartDockedFabLocation();
/// Start-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar,
/// optimized for mini floating action buttons.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.leading] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 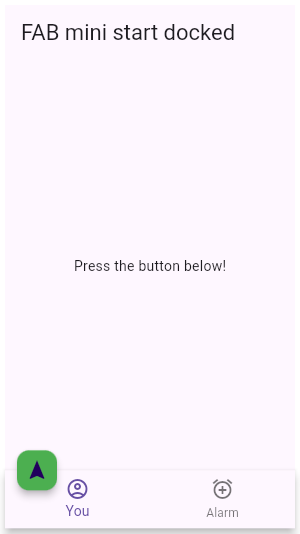
static const FloatingActionButtonLocation miniStartDocked = _MiniStartDockedFabLocation();
/// Centered [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 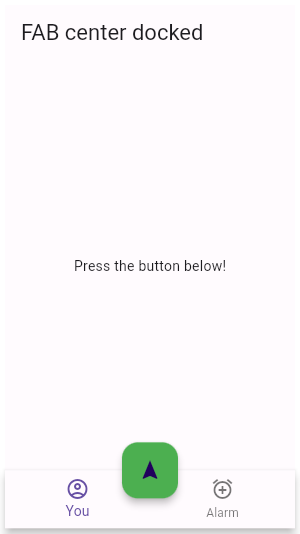
static const FloatingActionButtonLocation centerDocked = _CenterDockedFabLocation();
/// Centered [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar;
/// intended to be used with [FloatingActionButton.mini] set to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 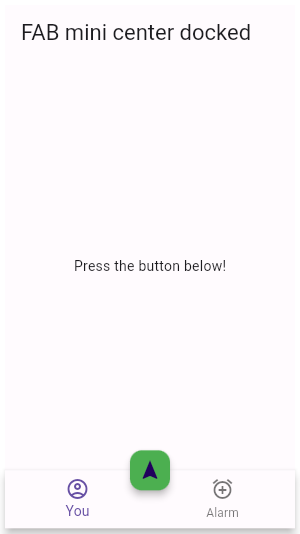
static const FloatingActionButtonLocation miniCenterDocked = _MiniCenterDockedFabLocation();
/// End-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 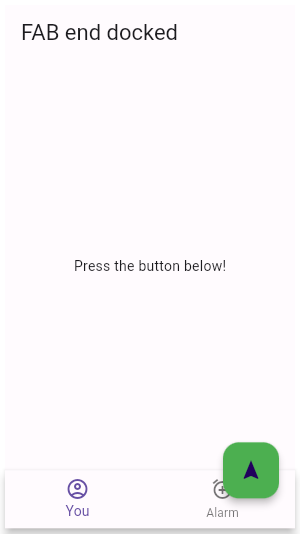
static const FloatingActionButtonLocation endDocked = _EndDockedFabLocation();
/// End-aligned [FloatingActionButton], floating over the
/// [Scaffold.bottomNavigationBar] so that the center of the floating
/// action button lines up with the top of the bottom navigation bar,
/// optimized for mini floating action buttons.
///
/// To align a floating action button with [CircleAvatar]s in the
/// [ListTile.trailing] slots of [ListTile]s in a [ListView] in the [Scaffold.body],
/// use [miniEndDocked] and set [FloatingActionButton.mini] to true.
///
/// If the value of [Scaffold.bottomNavigationBar] is a [BottomAppBar],
/// the bottom app bar can include a "notch" in its shape that accommodates
/// the overlapping floating action button.
///
/// This is intended to be used with [FloatingActionButton.mini] set to true,
/// so that the floating action button appears to align with [CircleAvatar]s
/// in the [ListTile.trailing] slot of a [ListTile] in a [ListView] in the
/// [Scaffold.body].
///
/// This is unlikely to be a useful location for apps that lack a bottom
/// navigation bar.
///
/// 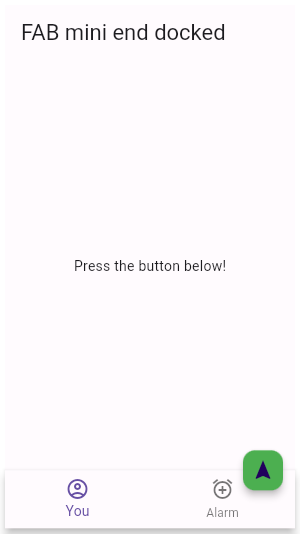
static const FloatingActionButtonLocation miniEndDocked = _MiniEndDockedFabLocation();
/// Places the [FloatingActionButton] based on the [Scaffold]'s layout.
///
/// This uses a [ScaffoldPrelayoutGeometry], which the [Scaffold] constructs
/// during its layout phase after it has laid out every widget it can lay out
/// except the [FloatingActionButton]. The [Scaffold] uses the [Offset]
/// returned from this method to position the [FloatingActionButton] and
/// complete its layout.
Offset getOffset(ScaffoldPrelayoutGeometry scaffoldGeometry);
String toString() => objectRuntimeType(this, 'FloatingActionButtonLocation');
}
FloatingActionButton WidgetをPaddingで囲う方法
FABのマージンを設定するためには、以下の通り、FloatingActionButton WidgetをPaddingで囲う。Paddingは、childの周りのPaddingを設定することになるため、結果的にFABのマージンとなる。
floatingActionButton: Padding(
padding: const EdgeInsets.only(bottom: 50.0),
child: FloatingActionButton(onPressed: () {}),
),
のように、bottomに対してpaddingを設定すれば、
とBannerAdを避けるようにしてpaddingを設定できる。
ただ、結局BannerAdとFABをまたいでの操作となり、UX的に良くないと考え、FABを横に避ける形でBannerAdを表示することとした。
Discussion