Kotlinの公式コーディング規約を読んでみた
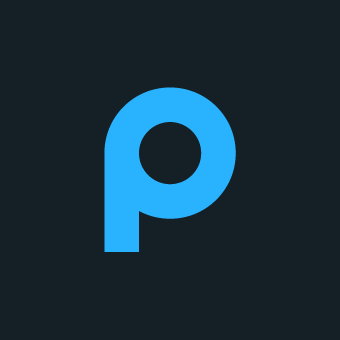
原文
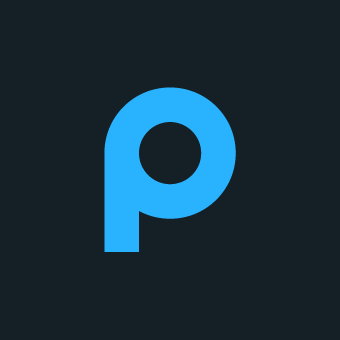
Directory structure
the recommended directory structure follows the package structure with the common root package omitted.
ディレクトリ構成はパッケージ構成と同じにすることが推奨されている
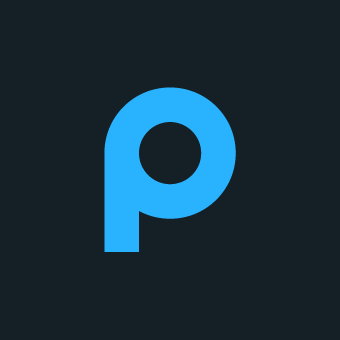
Source file names
Use upper camel case
アッパーキャメル形式のファイル名
If a Kotlin file contains a single class (potentially with related top-level declarations), its name should be the same as the name of the class
ファイルが一つのクラスしか含まない場合はクラス名と同じファイル名にしましょう
If a file contains multiple classes, or only top-level declarations, choose a name describing what the file contains
ファイルが複数のクラスを含む or トップレベル宣言で構成される場合は構成要素を表現した名前にしましょう
The name of the file should describe what the code in the file does. Therefore, you should avoid using meaningless words such as Util in file names.
ファイル名にはそのファイルが何をするのかを表現すべきでありUtil
のような意味のなさないファイル名はやめましょう
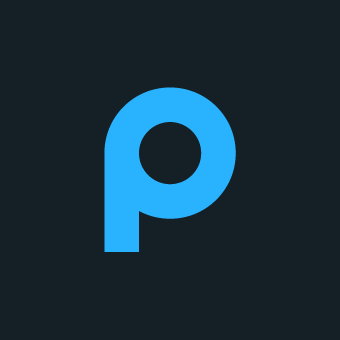
Source file organization
Placing multiple declarations (classes, top-level functions or properties) in the same Kotlin source file is encouraged as long as these declarations are closely related to each other semantically
ひとつのKotlinファイルのクラスやトップレベル関数、プロパティを書くのはそれぞれが、意味的に密に関連している場合に限って推奨されます
In particular, when defining extension functions for a class which are relevant for all clients of this class, put them in the same file with the class itself.
特に関連するすべてのクラスのための拡張関数を定義するような場合には同一ファイルに定義しましょう
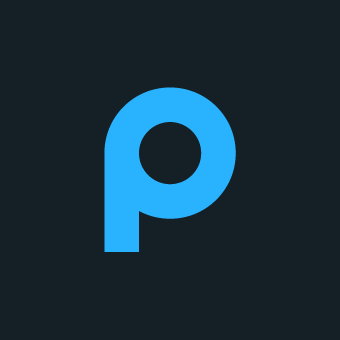
Class layout
The contents of a class should go in the following order:
Property declarations and initializer blocks
Secondary constructors
Method declarations
Companion object
クラス内の定義順
- プロパティ宣言, init関数
- セカンダリコンストラクタ
- メソッド
- companion object
Do not sort the method declarations alphabetically or by visibility, and do not separate regular methods from extension methods. Instead, put related stuff together
アルファベット順や可視性による並び順ではなく、関連し合うもの同士を近くに置きましょう
(読み手が順番に読むときに何が起こるかわかりやすいよねってことみたい)
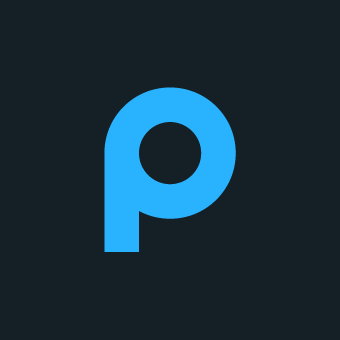
Interface implementation layout
When implementing an interface, keep the implementing members in the same order as members of the interface
インターフェースの実装クラスはインターフェースで定義された順にメソッドを並べましょう
(privateメソッドはいろいろな場所に挿入してもOK)
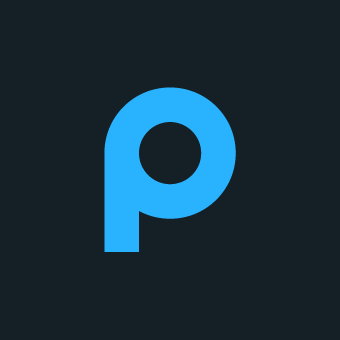
Overload layout
Always put overloads next to each other in a class.
オーバーロードしたメソッドは元のメソッドの次に並べていきましょう
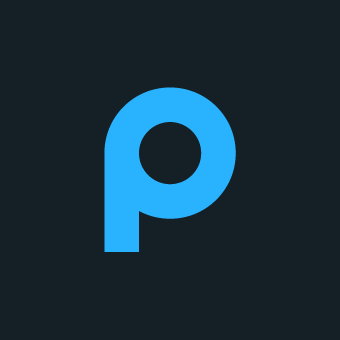
Package and class naming rules
Names of packages are always lowercase and do not use underscores. Using multi-word names is generally discouraged, but if you do need to use multiple words, you can either just concatenate them together or use camel case
パッケージ名はすべて小文字、複数単語は極力使わない。使わなくてはならない場合はそのまま小文字で繋げるかキャメルケースにする
Names of classes and objects start with an uppercase letter and use camel case:
クラス名(オブジェクト名)はアッパーキャメルケース
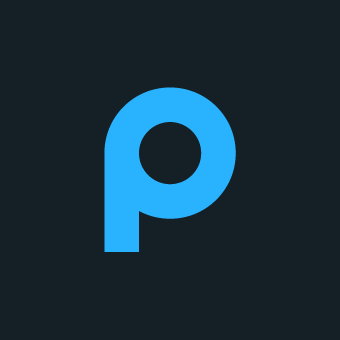
Function names
Names of functions, properties and local variables start with a lowercase letter and use camel case and no underscores:
関数名・プロパティ名、ローカル変数名はキャメルケースでアンダースコアは使わない
Exception: factory functions used to create instances of classes can have the same name as the abstract return type:
(例外)抽象を返すファクトリ関数は抽象クラス(インターフェース)名と同じにする
interface Hoge { /*...*/ }
class HogeImpl : Hoge { /*...*/ }
fun Hoge(): Hoge { return HogeImpl() }
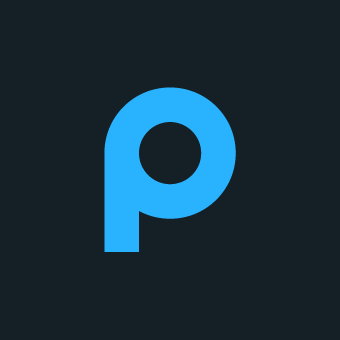
Names for test methods
In tests (and only in tests), you can use method names with spaces enclosed in backticks.
テストソースに限ってスペースを使ってもいいよ
@Test fun `ensure everything works`() { /*...*/ }
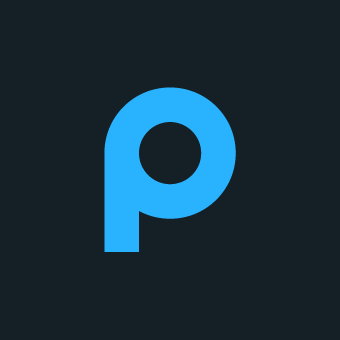
Property names
Names of constants (properties marked with const, or top-level or object val properties with no custom get function) should use uppercase underscore-separated
- const 宣言
- トップレベルに宣言されたvalプロパティ
- objectのvalプロパティ(※但しカスタムgetterを持たないもの)
これら(真にイミュータブルなもの)は大文字のアンダースコア区切りが望ましい
Names of top-level or object properties which hold objects with behavior or mutable data should use camel case names:
前述のものでも振る舞いを持つあるいは、ミュータブルなものはキャメルケースが望ましい
Names of properties holding references to singleton objects can use the same naming style as object declarations:
シングルトンなものはobjectの規約に従う
For enum constants, it's OK to use either uppercase underscore-separated names
Enumは大文字かつアンダースコア区切り使ってOK
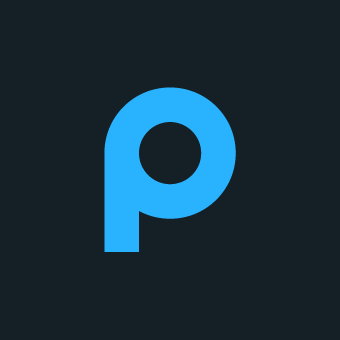
Names for backing properties
use an underscore as the prefix for the name of the private property
バッキングフィールドはアンダースコアで始めなさい
class Person {
private val _text: String = ""
val text: String
get() {
return _text
}
}
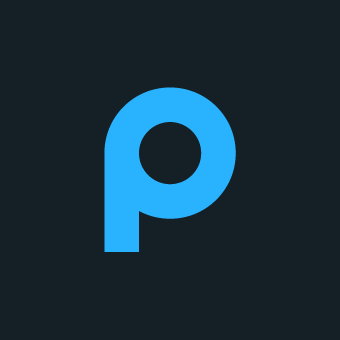
Choose good names
The name of a class is usually a noun
クラス名はたいてい名詞系
The name of a method is usually a verb
メソッド名はたいてい動詞系
The name should also suggest if the method is mutating the object or returning a new one
副作用のあるメソッドはそうであることが伝わる名称にしよう
- sort => 対象のインスタンスを直接ソートする
- sorted => 対象のインスタンスをソートした新しいインスタンスが返る
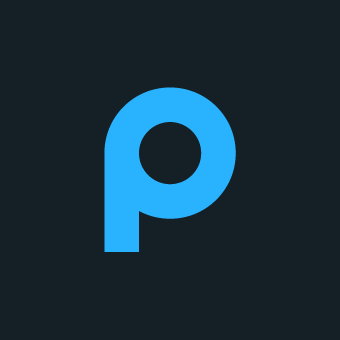
Indentation
Use four spaces for indentation. Do not use tabs.
スペースで4つ分
put the opening brace in the end of the line where the construct begins
// OK
fun some() {
}
// NG
fun some()
{
}
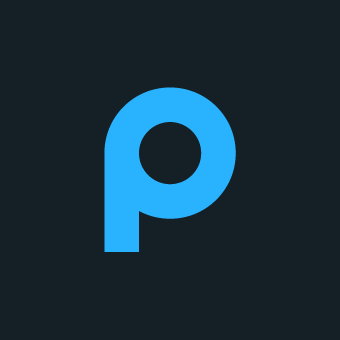
Horizontal whitespace(※一部)
Put spaces between control flow keywords (if, when, for, and while)
if, when, for, whileの後はスペース入れなさい
Never put a space after (, [, or before ], )
(,[の後、],)の前にはスペースは入れない
Put a space after //: // This is a comment
// の後にはスペースを入れなさい
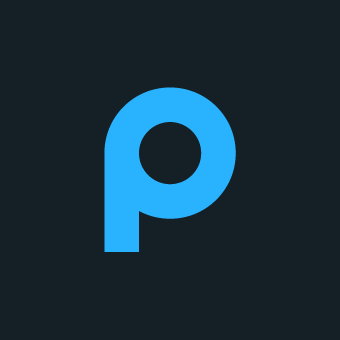