Open5
SwiftUI
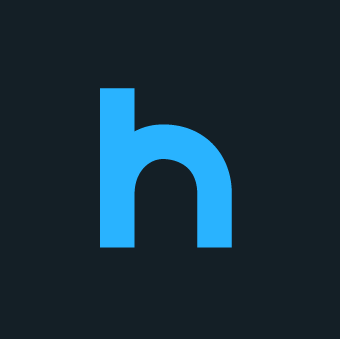
addCurve(to:controlPoint1:controlPoint2:)
Appends a cubic Bézier curve to the path.
func addCurve(to endPoint: CGPoint,
controlPoint1: CGPoint,
controlPoint2: CGPoint)
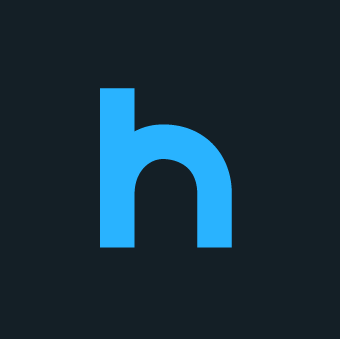
Extension for adding rounded corners in specific corners
// Extension for adding rounded corners in specific corners
extension View {
func cornerRadisu(_ radius: CGFloat, corners: UIRectCorner) -> some View {
clipShape(RoundedCorner(radius: radius, corners: corners))
}
}
// Custom RoundedCorner shape used for cornerRadius extension above
struct RoundedCorner: Shape {
var radius: CGFloat = .infinity
var corners: UIRectCorner = .allCorners
func path(in rect: CGRect) -> Path {
let path = UIBezierPath(roundedRect: rect, byRoundingCorners: corners, cornerRadii: CGSize(width: radius, height: radius))
return Path(path.cgPath)
}
}
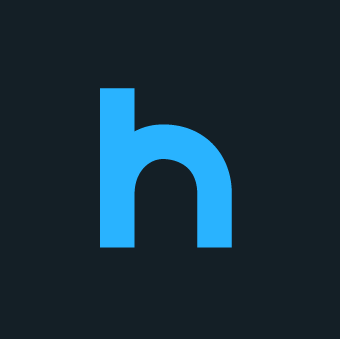
ScrollViewReader
The scroll view reader’s content view builder receives a ScrollViewProxy instance; you use the proxy’s scrollTo(_:anchor:) to perform scrolling.
@Namespace var topID
@Namespace var bottomID
var body: some View {
ScrollViewReader { proxy in
ScrollView {
Button("Scroll to Bottom") {
withAnimation {
proxy.scrollTo(bottomID)
}
}
.id(topID)
VStack(spacing: 0) {
ForEach(0..<100) { i in
color(fraction: Double(i) / 100)
.frame(height: 32)
}
}
Button("Top") {
withAnimation {
proxy.scrollTo(topID)
}
}
.id(bottomID)
}
}
}
func color(fraction: Double) -> Color {
Color(red: fraction, green: 1 - fraction, blue: 0.5)
}
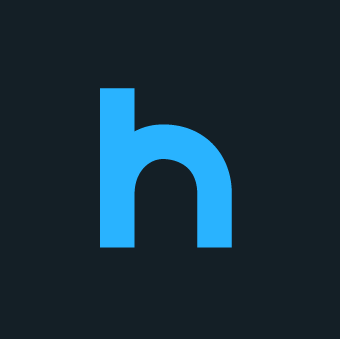
Codable
let menuList = documents.compactMap { doc in
let result = Result {
try doc.data(as: Menu.self)
}
switch result {
case .success(let _menu):
if let menu = _menu {
return menu
} else {
print("Document does not exist")
return nil
}
case .failure(let error):
print("Error decoding menu: \(error.localizedDescription)")
return nil
}
}
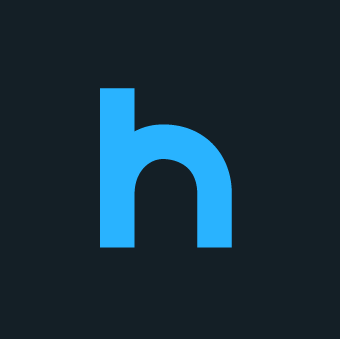
CaseIterable
- A type that provides a collection of all of its values.
4.2以前
enum Direction1 {
case east
case west
case north
case south
static var allCases: [Direction1] = [.east, .west, .north, .south]
}
4.2以降
enum Direction2: CaseIterable {
case east
case west
case north
case south
}