openapi-typescriptのCLIオプション、全部見る
はじめに
openapi-typescript は OpenAPI 3.0,3.1 のスキーマを TypeScript に変換するツールです。
以下のようにスキーマファイルのパスと出力ファイルのパスを指定することで、スキーマを TS に変換できます。
npx openapi-typescript ./path/to/my/schema.yaml -o ./path/to/my/schema.ts
CLI オプションというのは、上記例の「-o」のように、スキーマから TS に変換する際の振る舞いを制御できる仕組みです.
この記事では、openapi-typescript で使用できるオプションがどのようなものがあるかを見ていきます。
オプション一覧
出力に関するオプション
flag | alias | default | description |
---|---|---|---|
--output [location] |
-o |
(stdout) | 出力ファイルを保存する場所を指定します |
--alphabetize |
false | 型をアルファベット順にソートします |
--output [location]
[location] (出力ファイル先) に生成した結果が出力されます。
/**
* This file was auto-generated by openapi-typescript.
* Do not make direct changes to the file.
*/
export interface paths { /* 実際の結果が入る */ }
export interface components { /* 実際の結果が入る */ }
export interface operations { /* 実際の結果が入る */ }
...
--alphabetize
型をアルファベット順にソートします
components:
schemas:
Beta:
type: object
properties:
b:
type: boolean
c:
type: boolean
a:
type: boolean
Alpha:
type: object
properties:
z:
type: boolean
a:
type: boolean
Gamma:
type: object
properties:
10:
type: boolean
2:
type: boolean
1:
type: boolean
export interface components {
schemas: {
Alpha: {
a?: boolean;
z?: boolean;
};
Beta: {
a?: boolean;
b?: boolean;
c?: boolean;
};
Gamma: {
1?: boolean;
2?: boolean;
10?: boolean;
};
};
上の例から分かる通り、localeCompare で Numeric sortingするので numeric な key も昇順にソートします。
if (options?.alphabetize) {
entries.sort(([a], [b]) => a.localeCompare(b, "en-us", { numeric: true }));
}
型の生成に関するオプション
flag | alias | default | description |
---|---|---|---|
--additional-properties |
false |
additionalProperties: false が指定されていない全てのスキーマオブジェクトに任意のプロパティを許可します |
|
--default-non-nullable |
true | default 値が設定されているスキーマオブジェクトを非 null として扱います(パラメータを除く) | |
--properties-required-by-default |
false |
required が指定されていないスキーマオブジェクトは全てのプロパティが必須であるとみなします |
|
--empty-objects-unknown |
false | プロパティが指定されておらず、additionalProperties も指定されていないスキーマオブジェクトに任意のプロパティを許可します |
|
--enum |
false | 文字列のユニオンではなく、Enum を生成します | |
--enum-values |
false | Enum の値を配列でエクスポートします | |
--export-type |
-t |
false |
interface の代わりにtype をエクスポートします |
--immutable |
false | immutable types を生成します(読み取り専用プロパティと読み取り専用配列) | |
--path-params-as-types |
false | paths オブジェクトでスキーマに記載された静的な URL に一致させる必要がなくなり、URL に基づいた動的な型参照が可能になります | |
--array-length |
false | 配列の minItems / maxItems を使用してタプルを生成します | |
--exclude-deprecated |
false | 型から非推奨フィールドを除外します |
--additional-properties
additionalProperties: false
が指定されていない全てのスキーマオブジェクトに任意のプロパティを許可します
export interface components {
schemas: {
animal: {
kind?: string;
};
};
}
export interface components {
schemas: {
animal: {
kind?: string;
[key: string]: unknown;
};
};
}
--default-non-nullable
default 値が設定されているスキーマオブジェクトを非 null として扱います(パラメータを除く)
export interface components {
schemas: {
animal: {
/** @default cat */
kind?: string;
};
};
}
export interface components {
schemas: {
animal: {
/** @default cat */
kind: string;
};
};
}
--properties-required-by-default
required
が指定されていないスキーマオブジェクトは全てのプロパティが必須であるとみなします
export interface components {
schemas: {
animal: {
id?: number;
kind?: string;
};
};
}
export interface components {
schemas: {
animal: {
id: number;
kind: string;
};
};
}
--empty-objects-unknown
OpenAPI スキーマの空のオブジェクトに対する型の扱いが変わります。
プロパティが指定されておらず、additionalProperties も指定されていないスキーマオブジェクトに任意のプロパティを許可します。
components:
schemas:
EmptyObject:
type: object # プロパティが存在しない
export interface components {
schemas: {
EmptyObject: Record<string, never>;
};
}
export interface components {
schemas: {
EmptyObject: Record<string, unknown>;
};
}
--enum
文字列のユニオンではなく、Enum を生成します
export interface components {
schemas: {
animal: {
/** @enum {string} */
kind?: "dog" | "cat";
};
};
export interface components {
schemas: {
animal: {
/** @enum {string} */
kind?: AnimalKind;
};
};
}
export enum AnimalKind {
dog = "dog",
cat = "cat"
}
--enum-values
Enum の値を配列でエクスポートします
export const animalKindValues: ReadonlyArray<
components["schemas"]["animal"]["kind"]
> = ["dog", "cat"];
--export-type
interface
の代わりにtype
をエクスポートします
export interface components { ... }
export type components = { ... }
--immutable
immutable types を生成します(読み取り専用プロパティと読み取り専用配列)
export interface components {
a: string;
b: string;
}
export interface components {
readonly a: string;
readonly b: string;
}
--path-params-as-types
公式ドキュメントに詳細があるので省略します。
--array-length
公式ドキュメントに詳細があるので省略します。
--exclude-deprecated
型から非推奨フィールドを除外します
schemas:
Alpha:
type: object
properties:
a:
type: boolean
deprecated: true
z:
type: boolean
schemas: {
Alpha: {
z?: boolean;
};
};
その他のオプション
flag | alias | default | description |
---|---|---|---|
--help |
ヘルプメッセージを表示して終了します | ||
--version |
ライブラリのバージョンを表示して終了します | ||
--check |
false | 生成された型が最新であることを確認します | |
--redocly [location] |
redocly.yaml ファイルのパスを指定します |
--help
以下の通り ヘルプ情報(Usage と Options) が表示されます。
Usage
$ openapi-typescript [input] [options]
Options
--help Display this
--version Display the version
--redocly [path], -c Specify path to Redocly config (default: redocly.yaml)
--output, -o Specify output file (if not specified in redocly.yaml)
--enum Export true TS enums instead of unions
--enum-values Export enum values as arrays
--check Check that the generated types are up-to-date. (default: false)
--export-type, -t Export top-level `type` instead of `interface`
--immutable Generate readonly types
--additional-properties Treat schema objects as if `additionalProperties: true` is set
--empty-objects-unknown Generate `unknown` instead of `Record<string, never>` for empty objects
--default-non-nullable Set to `false` to ignore default values when generating non-nullable types
--properties-required-by-default
Treat schema objects as if `required` is set to all properties by default
--array-length Generate tuples using array minItems / maxItems
--path-params-as-types Convert paths to template literal types
--alphabetize Sort object keys alphabetically
--exclude-deprecated Exclude deprecated types
--version
使用している openapi-typescript のバージョンが表示されます。
v7.1.0
--check
生成された型が最新であることを確認します。最新でない場合は、以下のようにエラーが表示されます。
✘ Generated types are not up-to-date!
--redocly [location]
redocly.yaml ファイルのパスを指定します。
おわりに
筆者は基本的にはデフォルトのまま使用することが多いですが、こうしてみると色々なオプションがあることを再確認できました。何かの参考になれば嬉しいです。
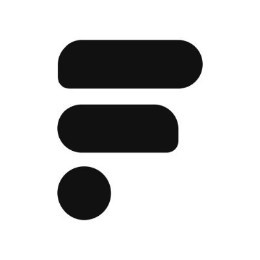
ユーザーファーストなサービスを伴に考えながらつくる、デザインとエンジニアリングの会社です。エンジニア積極採用中です!hrmos.co/pages/funteractive/jobs
Discussion