【ケモインフォマティクス事例】pubchemのapiで類似する化合物の取得する
pubchemのapiを使用すると化合物のfingerprint使用して類似する化合物を取得することができます。
化合物のfingerprint(指紋)は、化学的な構造情報を数値ベクトルまたはバイナリコードの形式で表現したもので、化学情報学や薬物デザインなどの分野で広く使用されています。これは、化合物の類似性評価、データベース検索、薬物デザイン、薬物発見などのさまざまな用途に役立つツールです。
化合物のfingerprintは通常、以下のようなプロセスを通じて生成されます:
1. 分子の表現: まず、対象の化合物が分子構造として表現されます。これは、元素や結合の情報を含む化学式や、原子の座標情報を含む三次元構造として表現されることがあります。
2. 部分構造の抽出: 化合物の構造から、特定の部分構造(例:官能基、環状構造、置換基)が抽出されます。これらの部分構造は、化合物内の特定の機能性や特性を示します。
3. 指紋の生成: 部分構造の情報をもとに、化合物の指紋が生成されます。指紋は通常、バイナリ(0または1)のベクトルとして表現されます。各要素は、化合物が特定の部分構造を持つ場合に1を示し、持たない場合に0を示します。指紋のサイズは、部分構造の数や指紋の精度によって異なります。
4. 指紋の利用: 生成された指紋は、データベース内の他の化合物との類似性を比較するために使用されます。2つの化合物の指紋が類似している場合、これらの化合物は化学的に関連性が高いとみなされます。また、指紋を用いて化合物のクラスタリング、機械学習モデルのトレーニング、薬物スクリーニングなど、さまざまなタスクにも利用されます。
- 指紋は、化合物の高速な比較と検索に役立つため、薬物設計や医薬品発見において重要なツールです。指紋ベースの類似性評価は、新しい候補薬の特性を予測するためにも使用され、効率的な化学空間の探索に寄与しています。指紋の生成方法や利用法は多岐にわたり、化学情報学の分野で継続的に研究されています。
関数
import requests, time, traceback
import numpy as np
def pubchemPropCID(cid):
url_pug = f'https://pubchem.ncbi.nlm.nih.gov/rest/pug_view/data/compound/{cid}/JSON/?response_type=display'
response_pug = requests.get(url_pug)#, headers=HEADERS)
if response_pug.status_code != 200:
return {}
data = response_pug.json()
props = {}
for section in data['Record']['Section']:
if section['TOCHeading'] == 'Names and Identifiers':
for item2 in section['Section']:
if item2['TOCHeading'] == 'Other Identifiers':
for item3 in item2['Section']:
if item3['TOCHeading'] == 'CAS':
props['CAS'] = item3['Information']
if section['TOCHeading'] == 'Chemical and Physical Properties':
for subsection in section['Section']:
if subsection['TOCHeading'] == 'Experimental Properties':
for row in subsection['Section']:
if row['TOCHeading'] == 'Boiling Point':
props['Boiling Point'] = row['Information']
elif row['TOCHeading'] == 'Melting Point':
props['Melting Point'] = row['Information']
elif row['TOCHeading'] == 'Dissociation Constants':
props['Dissociation Constants'] = row['Information']
elif row['TOCHeading'] == 'LogP':
props['LogP'] = row['Information']
elif row['TOCHeading'] == 'Density':
props['Density'] = row['Information']
elif row['TOCHeading'] == 'Ionization Potential':
props['Ionization Potential'] = row['Information']
return props
def pubchemCompound(identifier, namespace='smiles', property=True, url_option=None, ERROR=False):
url = f'https://pubchem.ncbi.nlm.nih.gov/rest/pug/compound/{namespace}/{identifier}/JSON'
if url_option:
url += f'?{url_option}'
response = requests.get(url)
data = response.json()
if not 'PC_Compounds' in data:
return []
compounds = []
for compound in data['PC_Compounds']:
time.sleep(1.5)
try:
cid = compound['id']['id']['cid']
props = {}
for prop in compound['props']:
urn = prop['urn']
value = prop['value']
if urn['label'] == 'Fingerprint':
props['Fingerprint'] = value['binary']
elif urn['label'] == 'LogP':
props['CompoundLogP'] = value['fval']
elif urn['label'] == 'Molecular Weight':
props['MolecularWeight'] = value['sval']
elif urn['label'] == 'SMILES':
props['SMILES'] = value['sval']
elif urn['label'] == 'Log P':
props['XLogP'] = value['fval']
if property:
try:
props.update(pubchemPropCID(cid))
except:
if ERROR:
traceback.print_exc()
pass
compounds.append({'cid': cid, 'props': props})
except Exception as e:
pass
return compounds
def similarityCids(smiles:str, threshold:int):
url = f"https://pubchem.ncbi.nlm.nih.gov/rest/pug/compound/fastsimilarity_2d/smiles/{smiles}/cids/JSON?Threshold={threshold}"
r = requests.get(url)
r.raise_for_status()
cids = r.json()['IdentifierList']['CID']
return cids
実装
smiles = 'CC(=O)Oc1ccccc1C(=O)O'
threshold = 99
cids = similarityCids(smiles, threshold)
cidsの中身
[2244,
135269,
71586929,
95938,
3055063,
...
76973140,
86068465,
102100677,
102100678,
102100679]
cidから物化性状を取得する
props = [pubchemCompound(cid, 'cid') for cid in cids]
# props >>
[[{'cid': 2244,
'props': {'Fingerprint': '00000371C0703800000000000000000000000000000000000000300000000000000000010000001A00000800000C04809800320E80000600880220D208000208002420000888010608C80C273684351A827B60A5E01108B98788C8208E00000000000800000000000000100000000000000000',
'XLogP': 1.2,
'MolecularWeight': '180.16',
'SMILES': 'CC(=O)OC1=CC=CC=C1C(=O)O',
'CAS': [{'ReferenceNumber': 2,
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 3,
'URL': 'https://commonchemistry.cas.org/detail?cas_rn=50-78-2',
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 8,
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 29,
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 33,
'Name': 'CAS',
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 34,
'Name': 'CAS',
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 35,
'Name': 'CAS',
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
{'ReferenceNumber': 37,
'Value': {'StringWithMarkup': [{'String': '50-78-2'}]}},
...
[{'cid': 102100679,
'props': {'Fingerprint': '00000371C0703800000000000000000000000000000000000000300000000000000000010000001A00000800000C04809800320E80000600880220D208000208002420000888010608C80C273684351A827B60A5E01108B98788C8208E00000000000800000000000000100000000000000000',
'XLogP': 1.2,
'MolecularWeight': '181.16',
'SMILES': 'CC(=[17O])OC1=CC=CC=C1C(=O)O'}}]]
上記のコードで取得できる物化性状
- Fingerprint
- XLogP
- MolecularWeight
- SMILES
- CAS
- Boiling Point
- Melting Point
- Density
- LogP
- Dissociation Constants
株式会社piponでは技術でお困りのことがある方はオンライン相談が可能です。
こちらから会社概要資料をDLできます!
お問い合わせ内容に「オンライン相談希望」とご記載ください。
株式会社piponでは定期的に技術勉強会を開催しています。
ChatGPT・AI・データサイエンスについてご興味がある方は是非、ご参加ください。
株式会社piponではChatGPT・AI・データサイエンスについて業界ごとの事例を紹介しています。ご興味ある方はこちらのオウンドメディアをご覧ください。
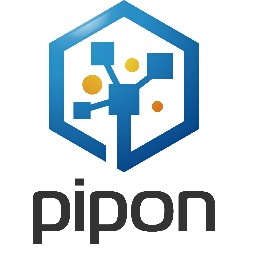
株式会社piponのテックブログです。 ChatGPTやAzureをメインに情報発信していきます! お問い合わせはフォームへお願いします。 会社HP pipon.co.jp/ フォーム share.hsforms.com/19XNce4U5TZuPebGH_BB9Igegfgt
Discussion