Angular+Springでサンプルアプリの作成
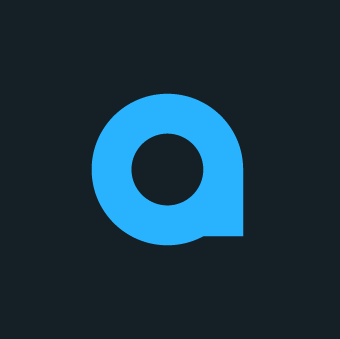
一覧画面の作成
APIからJsonデータを受け取り、画面表示する処理を実装する。
初期設定
まずはAngular CLIをインストール
npm install -g @angular/cli
CLIのインストール後はng
コマンドが使用可能になる。
プロジェクトはng new
コマンドから作成可能
ng new app-name
Goodsコンポーネントの生成
コンポーネントはng generate
から作成できる。
ng generate component goods
コマンドが完了すると、以下のファイルが格納されたgoods
フォルダが作成される。
- goods.component.ts
- goods.component.html
- goods.component.css
- goods.component.spec.ts
Angularではロジックとビューとスタイルが別ファイルに分けられている。
ロジックに相当するクラスファイルがgoods.component.ts
ビューに相当するのがgoods.component.html
スタイルに相当するのがgoods.component.css
生成されたgoods.component.ts
は以下のようになっている。
import { Component } from '@angular/core';
@Component({
selector: 'app-goods',
templateUrl: './goods.component.html',
styleUrls: ['./goods.component.css']
})
export class GoodsComponent {
}
selector
はCSS要素セレクタの設定。
これにより、親要素のビューで<app-goods>
と記述するとGoods
コンポーネントが呼び出せる。
templateUrl
はテンプレートファイルの場所を、styleUrls
はCSSスタイルの場所を定義している。
Goodsインターフェースの作成
export interface Order {
id: number;
name: string;
price: number;
}
GoodsServiceの作成
商品情報の取得処理はgoods
サービスで実装する。
ng generate service goods
import { Injectable } from '@angular/core';
import { Goods } from './goods';
@Injectable({
providedIn: 'root',
})
export class GoodsService {
goods: Goods[] = [];
getGoodsList() {
return this.goods;
}
}
Angularの依存性注入により、goods
サービスをコンストラクタで宣言することで使用できるようになる。
import { Component } from '@angular/core';
import { GoodsService } from '../goods.service';
@Component({
selector: 'app-goods',
templateUrl: './goods.component.html',
styleUrls: ['./goods.component.css']
})
export class GoodsComponent {
constructor(private goodsService: GoodsService) {}
}
## APIモックの作成
APIをモック化し、サーバーサイドからのデータ受け取りを疑似的に実装する。
`src/asset`配下にAPI作成後に受け取る予定のJsonファイルを作成する。
```json:src/asset/goods.json
[
{
"id": 1,
"name": "模造刀",
"price": 6000,
},
{
"id": 2,
"name": "ぬいぐるみ",
"price": 3000,
}
]
AngularのHttpClient
を使用し、goods.jsonから値を取得できるようにする。
HttpClient
を使用するためにはAppModule
にHttpClientModule
を追加する必要がある。
...
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [AppComponent, OrdersComponent],
imports: [
...
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
設定したらHttpClient
をインポートしコンストラクタで宣言。
getメソッドでJsonファイルのパスを指定し、ファイルからデータを取得するようにする。
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Goods } from './Goods';
@Injectable({
providedIn: 'root',
})
export class OrderService {
constructor(private http: HttpClient) {}
getOrders() {
return this.http.get<Order[]>('../asset/goods.json);
}
}
一覧画面の実装
一覧画面にはグリッド表示ライブラリであるag-grid
を使用する。
ag-grid-communityとag-grid-angularをインストールする。
npm install --save ag-grid-community
npm install --save ag-grid-angular
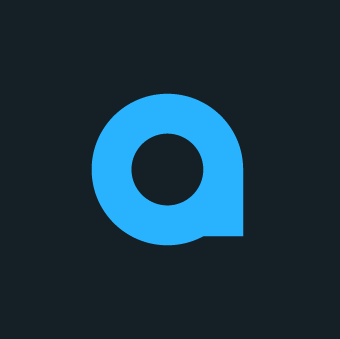
SpringでAPI作成
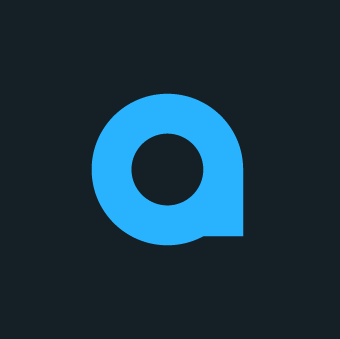